Semantic Kernel with C# minimal API
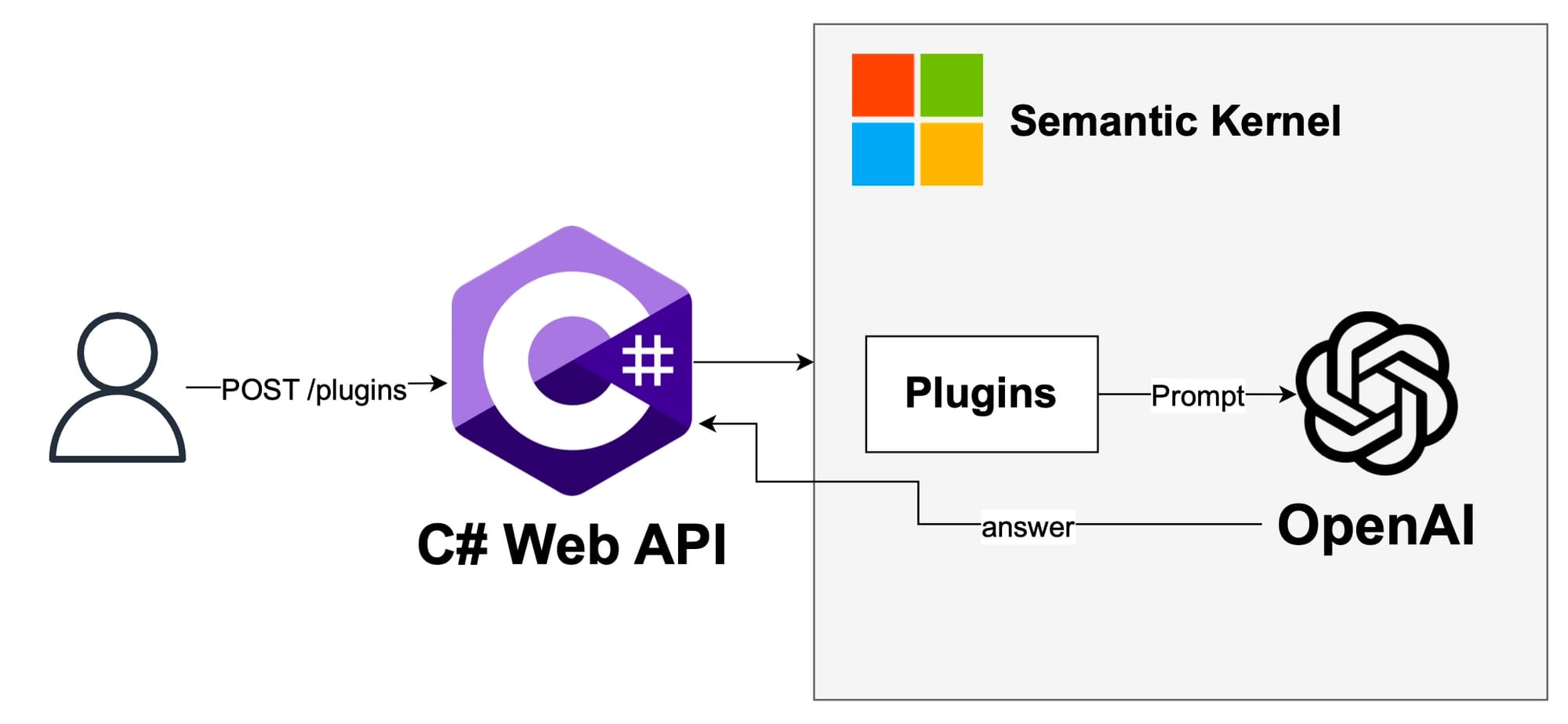
Overview
Are you keen on exploring the fusion of Artificial Intelligence (AI) and minimal APIs in the world of C#? If so, you're in the right place! In this article, I'll walk you through the integration of Semantic Kernel, an open-source SDK facilitating Large Language Models (LLMs) integration, into a minimal API built using ASP.NET Core in .NET 8. By using the power of OpenAI's GPT-3, we'll go on a journey to create a plugin that crafts narratives based on user input.
Introduction to Semantic Kernel and GPT-3
Recently I came across Semantic Kernel which is a fascinating open-source SDK that offers seamless integration with Large Language Models. I used the Semantic Kernel repo to get some inspiration. With a desire to incorporate AI capabilities into a C# project I decided to use GPT-3, an advanced language generation model developed by OpenAI. This powerful combination enables the creation of a minimal API leveraging the ASP.NET Core framework in .NET 8, setting the stage for crafting stories based on user inputs.
The AI plugins in Semantic Kernel do have a fixed structure. The config.json
is used to give instructions to OpenAI such as the temperature and max tokens. The skprompt.txt
is used to define a prompt. In my project I will use a prompt to instruct OpenAI to generate a story based on the user input and output the results as JSON.
Prerequisites
Before diving into the development process, ensure you have the following prerequisites in place:
- OpenAI Account and API Key: Obtain an API key from OpenAI to access GPT-3. Sign up for an account on the OpenAI platform if you haven't already.
- .NET 8 SDK: Install the .NET 8 Software Development Kit (SDK) to build and run the C# minimal API.
- Postman or another client to call the API.
- (Optional) Azure OpenAI subscription with an API key.
Getting Started with the Semantic Kernel Minimal API
To kickstart your journey with Semantic Kernel and the Minimal API, head over to the GitHub repository I've created. This repository houses the codebase you'll be working with throughout this tutorial.
Configuration
The application requires some request headers to be set by the client. In case of OpenAI a header called x-sk-web-app-key
is required. The value will be your generated API key from OpenAI.
Building and running the API
Run the following command in the terminal to launch the minimal API:
1dotnet build
2dotnet run
The API will start running locally, ready to receive requests.
Exploring the API Endpoints
Let's examine an example of how to use the API with Postman.
Example API Call
Make a POST request to http://localhost:5255/plugins/StoryPlugin/invoke/StoryGen
with the following JSON payload:
1{
2 "value": "A software developer, a plane to spain"
3}
Expected Response
1{
2 "list": [
3 "softwaredeveloper",
4 "plane",
5 "spain"
6 ],
7 "synopses": [
8 {
9 "tag": [
10 "softwaredeveloper"
11 ],
12 "chapter": {
13 "title": "The Life of a Software Developer",
14 "content": "Being a software developer involves creating, testing, and maintaining computer programs and applications. It requires skills in programming languages, problem-solving, and a deep understanding of technology. This chapter explores the daily life and challenges faced by software developers."
15 }
16 },
17 {
18 "tag": [
19 "plane"
20 ],
21 "chapter": {
22 "title": "Taking a Plane to Spain",
23 "content": "Traveling to Spain by plane is a convenient and efficient way to reach this beautiful country. This chapter covers the process of booking a flight, navigating airports, and the excitement of flying high above the clouds."
24 }
25 },
26 {
27 "tag": [
28 "spain"
29 ],
30 "chapter": {
31 "title": "Exploring the Wonders of Spain",
32 "content": "Spain is a vibrant country known for its rich history, delicious cuisine, and stunning architecture. This chapter delves into the various regions of Spain, highlights popular tourist attractions, and provides insights into the unique culture and traditions of the Spanish people."
33 }
34 }
35 ]
36}
Conclusion
Congratulations! You've successfully set up a minimal API integrating Semantic Kernel with GPT-3, allowing you to generate narrative snippets based on user input. This powerful combination of AI and C# opens doors to endless possibilities in creating AI-driven applications.
Explore the capabilities further, experiment with different inputs, and unlock the full potential of Semantic Kernel within your C# projects.
Feel free to contribute, share feedback, or expand upon this project by forking the repository and exploring its capabilities. Happy coding!